attribute和property的用法有何不同?
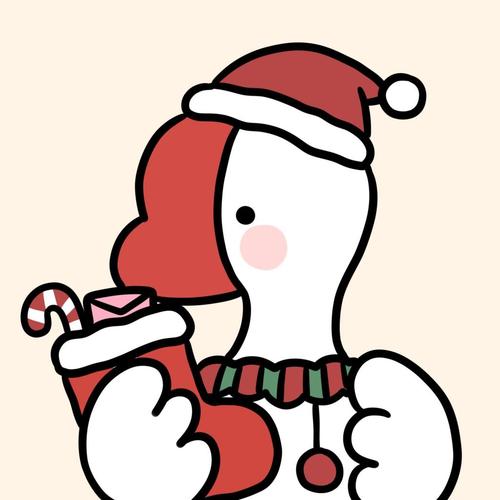
attribute和property的用法有何不同?
在编程中,我们经常会听到关于attribute和property的概念。这两个术语在不同的上下文中有不同的含义和用法。在本文中,我们将探讨attribute和property的区别和用法。
attribute是什么?
在编程中,attribute通常指的是对象的特性或特征。它描述了对象的状态或特定的特点。例如,在Python中,我们可以使用@attribute装饰器为类的属性添加额外的元数据。attribute可以是对象的内部数据,也可以是对象的外部元数据。它们通常是类的成员变量,用于存储对象的状态。
attribute的用途
attribute在编程中有许多用途。它们可以用于描述对象的状态,例如对象的颜色、大小、位置等。attribute还可以用于存储对象的配置信息或元数据。通过使用attribute,我们可以轻松地访问和修改对象的状态,以及在运行时动态地添加或删除attribute。
attribute的示例
以下是一个示例,展示了如何在Python中使用attribute:
```python
class Circle:
def __init__(self, radius):
self.radius = radius
@property
def area(self):
return 3.14 * self.radius * self.radius
circle = Circle(5)
print(circle.radius) 输出:5
print(circle.area) 输出:78.5
```
在上面的示例中,`radius`是Circle类的一个attribute,用于存储圆的半径。`area`是一个property,用于计算圆的面积。
property是什么?
在编程中,property通常指的是一种访问和修改对象属性的方式。它可以将一个方法转换为一个属性,从而让我们像访问普通的属性一样访问和修改它。property可以用于控制属性的读取和写入方式,以及对属性进行验证和计算。
property的用途
property在编程中有许多用途。它们可以用于隐藏属性的实现细节,使其看起来像普通的属性访问。property还可以用于对属性进行验证和计算,以确保属性的有效性和一致性。
property的示例
以下是一个示例,展示了如何在Python中使用property:
```python
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def radius(self):
return self._radius
@radius.setter
def radius(self, value):
if value <= 0:
raise ValueError("半径必须大于零")
self._radius = value
@property
def area(self):
return 3.14 * self._radius * self._radius
circle = Circle(5)
print(circle.radius) 输出:5
print(circle.area) 输出:78.5
circle.radius = 10
print(circle.radius) 输出:10
print(circle.area) 输出:314.0
circle.radius = -5 抛出ValueError异常
```
在上面的示例中,`radius`是一个property,用于访问和修改`_radius`属性。通过使用property,我们可以在设置半径时进行验证,并确保半径的有效性。
总结
attribute和property在编程中有不同的用途和含义。attribute描述了对象的特性或特征,用于存储对象的状态和元数据。property是一种访问和修改对象属性的方式,它可以将一个方法转换为一个属性,从而让我们像访问普通的属性一样访问和修改它。通过使用attribute和property,我们可以更好地组织和管理对象的数据和行为。
#编程 #Python #属性 #元数据